Appearance
question:Write a python function to determine if a list of numbers can represent a sequence of steps taken in a medical emergency situation. Each step is represented by a positive integer, and the sequence is considered valid if every number in the list is greater than the previous number. Your function should take a list of integers as input and return a boolean value: `True` if the sequence is valid, and `False` otherwise.Input: A list of integers, for example `[1, 3, 5, 7]`.Output: A boolean value, `True` if the sequence is strictly increasing, and `False` if it is not.Example:- Given the input `[1, 2, 3, 4]`, the function should return `True` because each number is greater than the previous one.- Given the input `[1, 3, 2, 4]`, the function should return `False` because `2` is not greater than `3`.
answer:Okay, I need to write a Python function to determine if a list of numbers represents a valid sequence of steps in a medical emergency. The condition is that each number must be greater than the previous one. So, the sequence has to be strictly increasing.Hmm, let's think about how to approach this. So, the function should take a list as input and return True or False based on whether each element is larger than the one before it.Wait, what if the list is empty? Or has just one element? Well, if the list is empty, maybe it's considered valid? Or perhaps the problem expects at least one step. But looking at the examples, the sample inputs have multiple elements. So perhaps, for a list with zero or one element, it's automatically valid. Because there's nothing to compare.So, the steps I need to take:1. Check if the list has less than two elements. If so, return True.2. Otherwise, iterate through the list starting from the second element.3. For each element, compare it with the previous one.4. If any element is not greater than the previous, return False.5. If all elements satisfy the condition, return True.Let me think about the edge cases.Case 1: Empty list. Should return True? Or maybe the problem expects at least one step. But the problem statement says each step is a positive integer, but the input is a list of integers. Wait, the problem says each step is a positive integer, but the input is a list of integers. So, perhaps the list can have zero or more elements. But for the sequence to be valid, each step must be greater than the previous. So, for a single-element list, it's trivially valid.Case 2: List with two elements. If the second is larger than the first, return True. Else, False.Another thing to consider: what if the list has negative numbers? Well, the problem says each step is a positive integer, but the function's input is a list of integers. So perhaps the function doesn't need to check for positivity, just the increasing order. Or maybe the function should also check that all numbers are positive? Wait, the problem statement says each step is represented by a positive integer, but the function's input is a list of integers. So, perhaps the function should first check if all elements are positive. Because, for example, if the list has a negative number, it's invalid regardless of the order.Wait, looking back at the problem statement: "Each step is represented by a positive integer, and the sequence is considered valid if every number in the list is greater than the previous number." So, the function needs to ensure two things: all numbers are positive, and each is greater than the previous.Oh right! I almost forgot that part. So, the function must first check that all numbers are positive. Because, for example, if the list is [2, 3, 4], it's valid. But if it's [0, 1, 2], then 0 is not a positive integer, so the sequence is invalid.Wait, wait. The problem says each step is a positive integer. So each number in the list must be positive, and each must be greater than the previous.So, the function needs to:- Check that every element in the list is a positive integer (greater than zero).- Check that each element is greater than the previous.So, the steps are:1. If the list is empty, return False? Because there are no steps. Or maybe, according to the problem statement, it's not a valid sequence if it's empty. Or perhaps, the function should return True for an empty list. Hmm, the problem says "a list of numbers can represent a sequence of steps". So, zero steps would be a valid sequence? Or not? Well, the problem's examples have non-empty lists. But the function's description says it takes a list of integers as input, which could be empty. So, perhaps the function should return True for an empty list because there's nothing wrong with it. Or maybe, the problem expects that a valid sequence must have at least one step, but the function's description says it can be any list.Wait, the problem says "each step is represented by a positive integer". So, if the list is empty, it's not a valid sequence because there are no steps. Or, perhaps it's considered valid as an empty sequence. Hmm, this is a bit ambiguous.But looking at the examples, the first example is [1,2,3,4], which is valid. The second example is [1,3,2,4], which is invalid. So, perhaps the function should return True for an empty list? Or maybe the function should return True only if the list is non-empty and all elements are positive and strictly increasing.Wait, the problem says, "a list of numbers can represent a sequence of steps taken in a medical emergency situation." So, the list must have at least one step, right? Because a medical emergency situation would involve at least one step. So, an empty list would not represent a valid sequence.So, the function should return True only if:- The list is non-empty.- Every element is a positive integer.- Each element is greater than the previous.Wait, but the function's input is a list of integers, which can include zero or negative numbers. So, the function needs to first check that all elements are positive.So, the function's logic:if the list is empty: return False.else: for each number in the list: if number <= 0: return False then, check if the list is strictly increasing. if yes: return True else: return FalseWait, but what about the case where the list has all positive numbers but is not strictly increasing? Like [1,2,2,3]. Then, it's invalid.So, the function should first check that all elements are positive, then check that each is larger than the previous.So, putting it all together.Now, how to implement this in Python.Function steps:def is_valid_sequence(numbers): # Check if the list is empty if not numbers: return False # Check all numbers are positive for num in numbers: if num <= 0: return False # Check if strictly increasing for i in range(1, len(numbers)): if numbers[i] <= numbers[i-1]: return False # If all checks passed return TrueWait, but what about a list with one element? Like [5]. Then, the function returns True, because it's non-empty, all elements are positive, and there's nothing to compare in the increasing check. So, it's valid.Yes, that's correct.Testing the examples:Example 1: [1,2,3,4] → all positive, each next is larger. So returns True.Example 2: [1,3,2,4] → 2 is not larger than 3. So returns False.Another test case: [5] → returns True.Another test case: [0,1,2] → 0 is not positive, returns False.Another test case: [-1, 2, 3] → -1 is not positive, returns False.Another test case: [2, 2, 3] → 2 is not larger than 2, returns False.Another test case: [] → returns False.Another test case: [3,4,5,6] → returns True.Another test case: [5,4,3,2,1] → returns False.So, the function should handle all these cases.Wait, but what about the case where the list has a single element which is negative? Like [-5]. Then, the function returns False because the number is not positive.Yes, that's correct.So, the function seems to cover all the necessary conditions.
question:Sister Maria, the young and charismatic nun, is preparing a lesson on compassion and tolerance using a mathematical analogy. She has a plan to use a quadratic equation to explain how different perspectives (solutions) can lead to understanding. Sister Maria introduces the concept to her students by presenting the following scenario:1. Sister Maria is organizing a school event, and she needs to arrange seating in a particular way. She wants to create a harmonious seating chart where the number of chairs in each row forms a quadratic pattern. If the number of chairs in a row can be represented by the equation ( x^2 + 5x + 6 = 0 ), how many chairs will be in each row?2. To ensure everyone feels included, Sister Maria also wants to create a small seating section with one more chair in each row than the previous arrangement. For this new arrangement, the number of chairs in each row can be represented by the equation ( x^2 + 6x + 8 = 0 ). How many chairs will be in each row in this new arrangement?Sister Maria hopes that by solving these equations, her students will learn not only about mathematics but also about looking at situations from different angles to find solutions.
answer:To solve the first quadratic equation ( x^2 + 5x + 6 = 0 ), I will factor it. I need two numbers that multiply to 6 and add up to 5. The numbers 2 and 3 satisfy these conditions, so the equation factors to ( (x + 2)(x + 3) = 0 ). Setting each factor equal to zero gives the solutions ( x = -2 ) and ( x = -3 ). Since the number of chairs cannot be negative, the valid solutions are 2 and 3 chairs per row.For the second equation ( x^2 + 6x + 8 = 0 ), I will also factor it. I need two numbers that multiply to 8 and add up to 6. The numbers 2 and 4 fit these criteria, so the equation factors to ( (x + 2)(x + 4) = 0 ). Setting each factor equal to zero gives the solutions ( x = -2 ) and ( x = -4 ). Again, since the number of chairs cannot be negative, the valid solutions are 2 and 4 chairs per row.
question:Write a python function to calculate the average rating of a list of classic European films given their individual ratings. The input should be a list of dictionaries, where each dictionary contains the keys `'title'` (a string representing the film's title) and `'rating'` (a float representing the film's rating from 0 to 10). The function should return a float representing the average rating rounded to two decimal places.Here is an example input and the expected output:Example Input:```pythonfilms = [ {"title": "La Dolce Vita", "rating": 8.5}, {"title": "8½", "rating": 8.3}, {"title": "The 400 Blows", "rating": 8.1}, {"title": "Breathless", "rating": 7.9}, {"title": "Bicycle Thieves", "rating": 8.3}]```Expected Output:```8.22```Please ensure that the function handles an empty list gracefully by returning `0.0`.
answer:Okay, I need to write a Python function to calculate the average rating of a list of classic European films. The input is a list of dictionaries, each with 'title' and 'rating'. The function should return the average rating rounded to two decimal places. Oh, and if the list is empty, return 0.0.Hmm, let's think about the steps. First, I need to get all the ratings from each dictionary in the list. Then, sum them up and divide by the number of films. But wait, what if the list is empty? I have to handle that case to avoid division by zero.So, the function will start by checking if the list is empty. If it is, return 0.0 immediately. Otherwise, proceed to calculate the average.How to extract the ratings? I can loop through each film in the list and collect the 'rating' values. Maybe using a list comprehension to make it concise. Like [film['rating'] for film in films].Once I have the list of ratings, sum them. Then, divide by the number of films, which is len(films). Then, round the result to two decimal places.Wait, how to round correctly? Using the round function with two decimal places. Like round(total / count, 2).Putting it all together:Function steps:1. Check if films is empty. If yes, return 0.0.2. Else, extract all ratings into a list.3. Calculate the sum of the ratings.4. Divide by the number of films to get the average.5. Round the average to two decimal places.6. Return the rounded average.Let me test this logic with the example input.Example Input:films = [ {"title": "La Dolce Vita", "rating": 8.5}, {"title": "8½", "rating": 8.3}, {"title": "The 400 Blows", "rating": 8.1}, {"title": "Breathless", "rating": 7.9}, {"title": "Bicycle Thieves", "rating": 8.3}]Sum of ratings: 8.5 + 8.3 is 16.8, plus 8.1 is 24.9, plus 7.9 is 32.8, plus 8.3 is 41.1. So total is 41.1. Number of films is 5. 41.1 /5 = 8.22. So the output is 8.22, which matches the expected output.What about an empty list? films = [] → return 0.0.What about a single film? Like films = [{'title': 'A', 'rating': 5.0}] → average is 5.0, rounded to two decimals is 5.0.What about when the average has more than two decimal places? Like sum is 10.0 for two films → 5.0. Or sum is 10.123 for two films → 5.0615 → rounded to 5.06.So the function should handle all these cases.Now, coding this.Function name: calculate_average_rating.Parameters: films.Inside the function:if not films: return 0.0ratings = [film['rating'] for film in films]total = sum(ratings)average = total / len(films)rounded_average = round(average, 2)return rounded_averageWait, but what if the films list is empty? The first condition handles that.Yes.Testing the code with the example:films = [...] as given.ratings = [8.5, 8.3, 8.1, 7.9, 8.3]sum is 8.5 +8.3 is 16.8, +8.1=24.9, +7.9=32.8, +8.3=41.1. 41.1 /5 =8.22. So function returns 8.22.Another test case: films = [{'rating': 10}, {'rating': 0}] → sum is 10, average 5.0.Another test case: films = [{'rating': 7.5}, {'rating': 8.5}] → sum 16, average 8.0.What about when the average is something like 8.225 → round to 8.22 or 8.23? Because round(8.225, 2) in Python rounds to 8.22, but wait, no, let me check.Wait, in Python, round(8.225, 2) is 8.22, because of how floating points are handled. Or is it 8.23? Wait, no, let me think. Wait, 8.225 is exactly halfway between 8.22 and 8.23. Python uses "bankers rounding", which rounds to the nearest even number in such cases. So 8.225 would round to 8.22, because the second decimal is 2, which is even.Wait, no, wait: 8.225 is 8.225. The third decimal is 5, so when rounding to two decimals, it's 8.23, right? Or does it depend on the implementation.Wait, perhaps I should test this. But regardless, the function is to return the average rounded to two decimals as per Python's round function.So the code is correct.So putting it all together, the function is:def calculate_average_rating(films): if not films: return 0.0 ratings = [film['rating'] for film in films] average = sum(ratings) / len(films) return round(average, 2)Wait, but the return type is a float. So for the case when the average is 8.22, it returns 8.22 as a float.Yes.Testing with the example, it should return 8.22.Another test case: films = [{'title': 'A', 'rating': 8.22}, {'title': 'B', 'rating': 8.23}]. Sum is 16.45, average is 8.225. Rounded to two decimals is 8.22 or 8.23? Let's see.Wait, 8.225 rounded to two decimals: the third decimal is 5, so it rounds up. So 8.225 → 8.23? Or does it round to even?Wait, 8.225 is exactly halfway between 8.22 and 8.23. So in Python, round(8.225, 2) would round to 8.22, because 2 is even. Let me check:Wait, no, wait: 8.225 is 8.225. The third decimal is 5. So when rounding to two decimals, the second decimal is 2. Adding 0.005 would make it 8.23. But in Python, the round function uses "bankers rounding", which rounds to the nearest even number when the number is exactly halfway between two options.So 8.225 → 8.22 because 2 is even.Wait, let me test in Python:>>> round(8.225, 2)8.22>>> round(8.235, 2)8.24Yes, that's correct. So the function would return 8.22 in that case.So the code is correct.Another test case: films is empty → returns 0.0.So the function should handle all cases correctly.
question:Takumi Watanabe is experimenting with a new signature cocktail recipe that involves blending different proportions of two types of special sake that he has sourced from different regions in Japan. The first type, Sake A, has an alcohol content of 15%, and the second type, Sake B, has an alcohol content of 20%. He aims to create a blend that will result in a cocktail with a 17% alcohol content.1. If Takumi wants to prepare a total of 5 liters of this cocktail blend, how many liters of Sake A and Sake B should he use to achieve the desired alcohol content?2. To add a unique twist, Takumi decides to incorporate a fruit syrup that dilutes the mixture's alcohol content by an additional 2%. If he adds 0.5 liters of this fruit syrup to the cocktail blend, how much of the original 5 liters of the sake mixture does he need to adjust (either increase or decrease) to maintain the final cocktail's alcohol content at 17% after adding the syrup?
answer:Alright, so I've got this problem about Takumi Watanabe mixing two types of sake to make a cocktail. There are two parts to it, and I need to figure out both. Let's start with the first one.**Problem 1:** He wants to make 5 liters of a cocktail that's 17% alcohol by mixing Sake A (15%) and Sake B (20%). I need to find out how much of each he should use.Hmm, okay. This sounds like a mixture problem where I have to find the right proportions. I remember that these kinds of problems can be solved using systems of equations. Let me think about how to set it up.Let's denote:- Let x be the liters of Sake A.- Let y be the liters of Sake B.We know that the total volume should be 5 liters, so:x + y = 5That's one equation. Now, for the alcohol content. The total alcohol from Sake A is 15% of x, which is 0.15x. The total alcohol from Sake B is 20% of y, which is 0.20y. The final mixture should be 17% alcohol of 5 liters, which is 0.17 * 5 = 0.85 liters of alcohol.So, the equation for the alcohol content is:0.15x + 0.20y = 0.85Now, I have two equations:1. x + y = 52. 0.15x + 0.20y = 0.85I can solve this system of equations. Maybe substitution would work here. From the first equation, I can express y in terms of x:y = 5 - xSubstitute this into the second equation:0.15x + 0.20(5 - x) = 0.85Let me compute that step by step.First, expand the equation:0.15x + 0.20*5 - 0.20x = 0.850.15x + 1 - 0.20x = 0.85Combine like terms:(0.15x - 0.20x) + 1 = 0.85-0.05x + 1 = 0.85Now, subtract 1 from both sides:-0.05x = 0.85 - 1-0.05x = -0.15Divide both sides by -0.05:x = (-0.15)/(-0.05)x = 3So, x is 3 liters. Then y is 5 - x = 5 - 3 = 2 liters.Wait, let me check that. If he uses 3 liters of Sake A (15%) and 2 liters of Sake B (20%), the total alcohol would be:3 * 0.15 = 0.45 liters2 * 0.20 = 0.40 litersTotal alcohol = 0.45 + 0.40 = 0.85 litersWhich is 17% of 5 liters (since 5 * 0.17 = 0.85). That checks out.So, for the first part, he needs 3 liters of Sake A and 2 liters of Sake B.**Problem 2:** Now, Takumi adds 0.5 liters of fruit syrup that dilutes the alcohol content by an additional 2%. He wants the final cocktail's alcohol content to remain at 17%. So, he needs to adjust the original 5 liters of sake mixture. I need to find out how much he should adjust—either increase or decrease—the original mixture.Hmm, okay. Let me try to understand this. The fruit syrup is being added to the cocktail, which dilutes the alcohol by 2%. So, the alcohol content after adding the syrup is 17% - 2% = 15%? Wait, no, that might not be the right way to think about it.Wait, the problem says the syrup dilutes the mixture's alcohol content by an additional 2%. So, if the original mixture is 17%, adding the syrup reduces it by 2%, making it 15%. But he wants the final alcohol content to still be 17%. So, he needs to compensate for the dilution by either adding more sake or perhaps adjusting the original mixture.Wait, let me parse the problem again: "If he adds 0.5 liters of this fruit syrup to the cocktail blend, how much of the original 5 liters of the sake mixture does he need to adjust (either increase or decrease) to maintain the final cocktail's alcohol content at 17% after adding the syrup?"So, the original 5 liters are the sake mixture (17% alcohol). Then, he adds 0.5 liters of syrup, which dilutes the alcohol by 2%. So, the alcohol content becomes 17% - 2% = 15%. But he wants it to stay at 17%. So, he needs to adjust the amount of the original mixture before adding the syrup so that when he adds the syrup, the alcohol content is still 17%.Alternatively, perhaps the syrup itself has 0% alcohol, and adding it dilutes the mixture. So, the total volume becomes 5 + 0.5 = 5.5 liters, and the alcohol content is reduced by 2%, so from 17% to 15%. But he wants it to remain at 17%, so he needs to adjust the original 5 liters.Wait, maybe I need to think in terms of the total alcohol before and after adding the syrup.Let me denote:- Let V be the adjusted volume of the original sake mixture (which is 17% alcohol).- He adds 0.5 liters of syrup, which I assume has 0% alcohol (since it's a syrup, maybe it's non-alcoholic? Or does it have some alcohol? The problem says it dilutes the mixture by 2%, so perhaps it's non-alcoholic. I think it's safe to assume the syrup is 0% alcohol unless stated otherwise.So, the total volume after adding syrup is V + 0.5 liters.The total alcohol is 0.17 * V (from the sake mixture) + 0 * 0.5 (from syrup) = 0.17V.He wants the final alcohol content to be 17%, so:(0.17V) / (V + 0.5) = 0.17Wait, that can't be right because if he adds non-alcoholic syrup, the alcohol content would decrease, but he wants it to stay the same. So, maybe he needs to increase the amount of alcohol by adjusting the original mixture.Wait, but the original mixture is 17%, so if he adds more of it, the total alcohol would increase, but the total volume would also increase. Alternatively, if he adds less of it, the total alcohol would decrease, but the total volume would also decrease.Wait, let me set up the equation properly.Let me denote:- Let x be the amount of the original 5 liters mixture he uses. So, he uses x liters of the 17% mixture and adds 0.5 liters of syrup.Total volume = x + 0.5 liters.Total alcohol = 0.17x + 0 = 0.17x.He wants the final alcohol content to be 17%, so:0.17x / (x + 0.5) = 0.17Wait, if I set this up, then:0.17x = 0.17(x + 0.5)Simplify:0.17x = 0.17x + 0.085Subtract 0.17x from both sides:0 = 0.085That can't be right. So, this suggests that it's impossible because adding non-alcoholic syrup would dilute the mixture, making the alcohol content less than 17%. Therefore, to maintain 17%, he must either add more alcohol or adjust the original mixture.Wait, but the original mixture is already 17%. So, if he adds syrup, which is 0%, the alcohol content will drop. To keep it at 17%, he needs to have more alcohol in the mixture before adding the syrup. So, perhaps he needs to use more of the original mixture, but that would just increase the total volume. Alternatively, maybe he needs to adjust the proportions of Sake A and Sake B in the original mixture to make it stronger before adding the syrup.Wait, but the problem says he needs to adjust the original 5 liters of the sake mixture. So, perhaps he needs to either increase or decrease the amount of the original mixture before adding the syrup so that when he adds the syrup, the final alcohol is 17%.Wait, let's think about it differently. Let me denote:Let V be the adjusted volume of the original mixture (which is 17% alcohol). He adds 0.5 liters of syrup (0% alcohol). The total volume is V + 0.5 liters. The total alcohol is 0.17V.He wants the final alcohol content to be 17%, so:0.17V / (V + 0.5) = 0.17But as before, this leads to 0.17V = 0.17(V + 0.5), which simplifies to 0 = 0.085, which is impossible. Therefore, the only way to achieve 17% alcohol after adding 0.5 liters of 0% syrup is to have the original mixture be stronger than 17%, so that when diluted, it reaches 17%.Wait, but the original mixture is 17%. So, perhaps he needs to adjust the original mixture by either adding more alcohol or using a stronger mixture.Wait, but the original mixture is fixed at 17%. So, maybe he needs to adjust the amount of the original mixture. Let me think again.Wait, perhaps the problem is that the syrup dilutes the mixture by 2%, meaning that the alcohol content is reduced by 2 percentage points, not 2% of the current content. So, if the original mixture is 17%, adding the syrup would make it 15%. But he wants it to stay at 17%, so he needs to have the mixture before adding syrup be 19%, so that when it's diluted by 2%, it becomes 17%.Wait, that might make sense. So, if the syrup reduces the alcohol content by 2 percentage points, then to end up at 17%, the mixture before adding syrup should be 19%.But the original mixture is 17%, so he needs to adjust it to 19%. How can he do that? By adding more of the stronger sake or less of the weaker sake.Wait, but the original mixture is 17%, which is a blend of Sake A (15%) and Sake B (20%). So, if he wants to make a stronger mixture (19%), he needs to use more of Sake B and less of Sake A.But the problem says he needs to adjust the original 5 liters of the sake mixture. So, perhaps he needs to either increase or decrease the amount of the original mixture before adding the syrup.Wait, maybe I'm overcomplicating it. Let me think of it as a new mixture where he adds syrup to the original mixture, and he needs to adjust the original mixture's volume so that the final alcohol is 17%.Let me denote:- Let V be the volume of the original 17% mixture he uses.- He adds 0.5 liters of syrup (0% alcohol).- Total volume = V + 0.5- Total alcohol = 0.17VHe wants the final alcohol content to be 17%, so:0.17V / (V + 0.5) = 0.17But as before, this leads to 0.17V = 0.17V + 0.085, which is impossible. Therefore, the only way is to have the original mixture be stronger, so that when diluted, it becomes 17%.So, let me denote:- Let C be the concentration of the original mixture before adding syrup.- After adding 0.5 liters of syrup, the concentration becomes C - 2% = 17%Therefore, C = 17% + 2% = 19%So, he needs the original mixture to be 19% alcohol. But the original mixture is 17%, so he needs to adjust it to 19%. How?He can do this by changing the proportions of Sake A and Sake B in the original mixture. Let's denote:- Let x be the liters of Sake A.- Let y be the liters of Sake B.- Total volume = x + y = V (which is the adjusted volume)- Total alcohol = 0.15x + 0.20y = 0.19VBut he also has to add 0.5 liters of syrup, so the total final volume is V + 0.5, and the total alcohol is 0.19V, which should be 17% of (V + 0.5):0.19V = 0.17(V + 0.5)Let me solve this equation.0.19V = 0.17V + 0.085Subtract 0.17V from both sides:0.02V = 0.085Divide both sides by 0.02:V = 0.085 / 0.02V = 4.25 litersSo, he needs to adjust the original mixture to 4.25 liters, which is 19% alcohol. Then, when he adds 0.5 liters of syrup, the total volume becomes 4.75 liters, and the alcohol content is 0.19 * 4.25 = 0.8075 liters, which is 0.8075 / 4.75 = 0.17 or 17%.So, he needs to adjust the original 5 liters to 4.25 liters. That means he needs to decrease the original mixture by 5 - 4.25 = 0.75 liters.Wait, but let me check this. If he uses 4.25 liters of the 19% mixture, which is made from Sake A and B, and adds 0.5 liters of syrup, the total is 4.75 liters. The alcohol is 0.19 * 4.25 = 0.8075 liters, which is 0.8075 / 4.75 = 0.17 or 17%. That works.But how does he make the 19% mixture? He needs to adjust the proportions of Sake A and B in the original mixture. Let's find out how much of each he needs to make 4.25 liters of 19% alcohol.Let me set up the equations again.Let x be liters of Sake A, y be liters of Sake B.x + y = 4.250.15x + 0.20y = 0.19 * 4.25Calculate 0.19 * 4.25:0.19 * 4 = 0.760.19 * 0.25 = 0.0475Total = 0.76 + 0.0475 = 0.8075 litersSo, equation 2: 0.15x + 0.20y = 0.8075From equation 1: y = 4.25 - xSubstitute into equation 2:0.15x + 0.20(4.25 - x) = 0.8075Compute:0.15x + 0.85 - 0.20x = 0.8075Combine like terms:-0.05x + 0.85 = 0.8075Subtract 0.85 from both sides:-0.05x = -0.0425Divide by -0.05:x = (-0.0425)/(-0.05) = 0.85 litersSo, x = 0.85 liters of Sake A, and y = 4.25 - 0.85 = 3.4 liters of Sake B.Wait, but originally, he was using 3 liters of A and 2 liters of B for 5 liters. Now, he's using 0.85 liters of A and 3.4 liters of B for 4.25 liters. So, he's decreasing the amount of A and increasing B, which makes sense because he needs a stronger mixture.But the question is asking how much of the original 5 liters of the sake mixture does he need to adjust. So, he was originally making 5 liters, but now he needs to make 4.25 liters. So, he needs to decrease the original mixture by 5 - 4.25 = 0.75 liters.Alternatively, if he wants to maintain the same proportions as before, but I don't think that's necessary because he needs a stronger mixture.Wait, but the problem says "how much of the original 5 liters of the sake mixture does he need to adjust (either increase or decrease) to maintain the final cocktail's alcohol content at 17% after adding the syrup."So, he was planning to use 5 liters of the 17% mixture, but now he needs to adjust it to 4.25 liters, so he needs to decrease it by 0.75 liters.Alternatively, if he wants to keep the total volume the same after adding syrup, he might need to adjust differently, but the problem doesn't specify that. It just says he adds 0.5 liters of syrup to the cocktail blend, so the total becomes 5 + 0.5 = 5.5 liters, but he wants the alcohol to still be 17%. But as we saw earlier, that's impossible because adding non-alcoholic syrup would dilute it. Therefore, he needs to adjust the original mixture to be stronger so that when he adds the syrup, the final alcohol is 17%.So, in conclusion, he needs to adjust the original mixture from 5 liters to 4.25 liters, which is a decrease of 0.75 liters.Wait, but let me double-check. If he uses 4.25 liters of 19% mixture and adds 0.5 liters of syrup, the total is 4.75 liters, and the alcohol is 0.8075 liters, which is 17%. So, yes, that works.Therefore, the answer is that he needs to decrease the original mixture by 0.75 liters.But wait, another way to think about it is that the syrup adds 0.5 liters, so the total volume becomes 5 + 0.5 = 5.5 liters. To have 17% alcohol, he needs 0.17 * 5.5 = 0.935 liters of alcohol.Originally, the 5 liters of mixture had 0.85 liters of alcohol. So, he needs an additional 0.935 - 0.85 = 0.085 liters of alcohol. But he can't add more alcohol, so he needs to adjust the original mixture to have more alcohol.Wait, but he can't add more alcohol; he can only adjust the amount of the original mixture. So, if he uses more of the original mixture, he gets more alcohol, but the total volume increases. Alternatively, if he uses less, he gets less alcohol, but the total volume decreases.Wait, this is getting confusing. Let me try another approach.Let me denote:- Let V be the adjusted volume of the original 17% mixture.- He adds 0.5 liters of syrup.- Total volume = V + 0.5- Total alcohol = 0.17V- He wants 0.17V / (V + 0.5) = 0.17But as before, this leads to 0.17V = 0.17(V + 0.5), which simplifies to 0 = 0.085, which is impossible. Therefore, the only way is to have the original mixture be stronger, so that when diluted, it becomes 17%.Therefore, the original mixture must be 19%, as before. So, he needs to make 4.25 liters of 19% mixture, which requires 0.85 liters of Sake A and 3.4 liters of Sake B, as calculated earlier.Therefore, he needs to adjust the original 5 liters to 4.25 liters, which is a decrease of 0.75 liters.So, the answer is that he needs to decrease the original mixture by 0.75 liters.Wait, but let me think again. If he uses 4.25 liters of the stronger mixture and adds 0.5 liters of syrup, the total is 4.75 liters with 0.8075 liters of alcohol, which is 17%. So, that works.Alternatively, if he wants to keep the total volume at 5 liters after adding syrup, he would need to adjust the original mixture to be 4.5 liters (since 4.5 + 0.5 = 5). But then, the alcohol would be 0.17 * 4.5 = 0.765 liters, and the final concentration would be 0.765 / 5 = 0.153 or 15.3%, which is less than 17%. So, that doesn't work.Therefore, the only way is to adjust the original mixture to 4.25 liters, which requires decreasing it by 0.75 liters.So, the answer to part 2 is that he needs to decrease the original mixture by 0.75 liters.**Final Answer**1. Takumi should use boxed{3} liters of Sake A and boxed{2} liters of Sake B.2. He needs to decrease the original mixture by boxed{0.75} liters.
question:In the diagram, ( AB ) and ( CD ) are straight lines.The value of ( x ) is(A) 50(B) 60(C) 70(D) 130(E) 230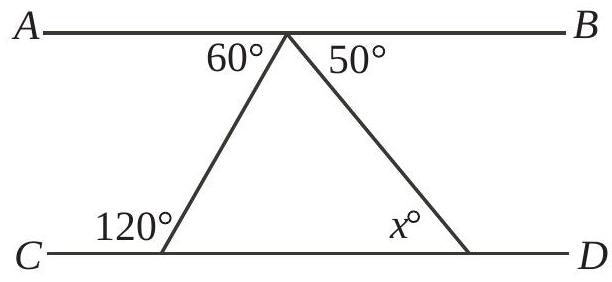
answer:Okay, so I have this geometry problem here with a diagram. The question is asking for the value of ( x ), and the options are 50, 60, 70, 130, and 230. Hmm, let me try to visualize the diagram based on the description. It says ( AB ) and ( CD ) are straight lines. I'm assuming that means they are straight lines intersecting or something. Maybe forming some angles?Since I can't see the diagram, I need to think about common geometry problems where two straight lines intersect and create angles, and we have to find an unknown angle ( x ). Maybe it's something with triangles, intersecting lines, or parallel lines with transversals.Wait, the options include 50, 60, 70, 130, and 230. Those are all different types of angles. 50, 60, 70 are acute angles, 130 is obtuse, and 230 is a reflex angle. So, depending on the diagram, ( x ) could be any of these. But since 230 seems quite large, maybe it's a reflex angle somewhere.Let me think about the possible scenarios. If ( AB ) and ( CD ) are straight lines, they could intersect at a point, forming vertical angles. If that's the case, then opposite angles would be equal. But without more information, it's hard to say.Alternatively, maybe ( AB ) and ( CD ) are parallel lines, and there's a transversal cutting through them, creating corresponding angles or alternate interior angles. If that's the case, then the angles would be equal or supplementary depending on their positions.Wait, another thought: maybe the diagram has triangles involved. If ( AB ) and ( CD ) are sides of triangles, then perhaps using triangle angle sum or exterior angle theorem could help.Since I don't have the exact diagram, I need to make some assumptions. Let me try to think of a common problem where two lines intersect, creating several angles, and one of them is ( x ). Maybe it's a problem involving supplementary angles, where two angles add up to 180 degrees.Alternatively, if there's a triangle with some given angles, and ( x ) is an exterior angle, then the exterior angle theorem could be used, which states that an exterior angle is equal to the sum of the two opposite interior angles.Wait, let me try to think of specific examples. If ( AB ) and ( CD ) intersect, forming vertical angles, and maybe some other lines creating triangles or other angles. Maybe there's a triangle with angles 50, 60, and 70, and ( x ) is one of those.Alternatively, maybe it's a problem with parallel lines and a transversal, so that corresponding angles are equal. For example, if one angle is 50 degrees, the corresponding angle would also be 50, and the alternate interior angle would be equal as well.Wait, another approach: since the options include 50, 60, 70, 130, and 230, perhaps ( x ) is part of a linear pair, meaning it adds up to 180 with another angle. So if one angle is 50, the adjacent angle would be 130, or if one is 60, the adjacent is 120, but 120 isn't an option. Hmm.Alternatively, maybe ( x ) is part of a triangle, and using the triangle angle sum, which is 180 degrees. So if two angles are given, say 50 and 60, then the third angle would be 70. So ( x ) could be 70.Wait, but without seeing the diagram, it's hard to be precise. Maybe I can think of another way. If ( AB ) and ( CD ) are straight lines, perhaps they form a quadrilateral, and we can use properties of quadrilaterals, like the sum of interior angles is 360 degrees.Alternatively, if it's a problem involving intersecting lines and some given angles, maybe ( x ) is an angle formed by those intersections.Wait, another idea: sometimes in these problems, you have angles around a point adding up to 360 degrees. So if there are several angles around a point, their sum is 360. So if some angles are given, ( x ) can be found by subtracting the sum of the known angles from 360.Alternatively, maybe it's a problem with angles on a straight line, which add up to 180 degrees. So if some angles are given on the line, ( x ) can be found by subtracting from 180.Wait, since 230 is an option, which is more than 180, that suggests that maybe ( x ) is a reflex angle, meaning it's greater than 180 degrees but less than 360. So if the diagram has a reflex angle, ( x ) could be 230.But how would that happen? Maybe if two lines intersect, creating several angles, and one of them is a reflex angle. For example, if one of the angles is 130 degrees, then the reflex angle would be 230, since 360 - 130 = 230.Alternatively, if ( x ) is an exterior angle of a triangle, it could be equal to the sum of the two non-adjacent interior angles. So if those two angles are, say, 50 and 60, then ( x ) would be 110, but that's not an option. Hmm.Wait, maybe it's a problem with parallel lines and a transversal, and ( x ) is an angle formed by that. For example, if one angle is 50 degrees, then the corresponding angle is also 50, and the consecutive interior angle would be 130, since they add up to 180.Alternatively, if it's an alternate interior angle, it would be equal. So if one angle is 60, the alternate interior angle is also 60.Wait, let me think step by step. Since the options are 50, 60, 70, 130, 230, and the lines are straight, maybe it's a problem where two lines intersect, creating vertical angles. So if one angle is 50, the opposite angle is also 50, and the adjacent angles would be 130 each, since 50 + 130 = 180.Alternatively, if it's a triangle with angles 50, 60, and 70, then ( x ) could be one of those. But without knowing which angle is which, it's hard to say.Wait, another thought: maybe it's a problem involving the exterior angle of a triangle. The exterior angle is equal to the sum of the two remote interior angles. So if the two remote interior angles are 50 and 60, then the exterior angle would be 110, which isn't an option. But if the two remote interior angles are 60 and 70, the exterior angle would be 130, which is an option.Alternatively, if the remote interior angles are 50 and 70, the exterior angle would be 120, which isn't an option. So maybe 130 is the exterior angle.Alternatively, if the diagram has a straight line with angles adding up to 180, and one angle is 50, then the adjacent angle would be 130, which is an option.Wait, but 230 is also an option. So maybe ( x ) is a reflex angle, which is 360 minus the smaller angle. So if the smaller angle is 130, the reflex angle would be 230.Alternatively, if the smaller angle is 50, the reflex angle would be 310, which isn't an option. If the smaller angle is 60, the reflex angle is 300, not an option. If it's 70, the reflex angle is 290, not an option. So only 130 would give a reflex angle of 230, which is an option.So maybe ( x ) is a reflex angle, which is 230, because the smaller angle is 130, and 360 - 130 = 230.Alternatively, maybe ( x ) is 130, which is supplementary to 50, since 50 + 130 = 180.Wait, but I need to figure out which one is more likely. Since 230 is an option, it's possible that ( x ) is a reflex angle. But without seeing the diagram, it's hard to know.Wait, another approach: maybe the diagram has two intersecting lines, creating four angles. If one of the angles is 50, then the opposite angle is also 50, and the adjacent angles are 130 each. So if ( x ) is one of the adjacent angles, it would be 130. If ( x ) is the reflex angle, it would be 230.Alternatively, maybe ( x ) is part of a triangle. If it's a triangle with angles 50, 60, and 70, then ( x ) could be 70. Alternatively, if it's an exterior angle, it could be 130.Wait, another thought: maybe it's a problem involving angles formed by two intersecting lines and a transversal. For example, if ( AB ) and ( CD ) are parallel lines cut by a transversal, creating corresponding angles. If one angle is 50, the corresponding angle is also 50, and the alternate interior angle is also 50. The consecutive interior angles would be 130 each, since they add up to 180.Alternatively, if the angle is 60, the consecutive interior angle would be 120, but that's not an option. So maybe 50 and 130 are the key angles here.Wait, let me think of a specific example. Suppose ( AB ) and ( CD ) are parallel lines, and a transversal cuts them, creating angles. If one of the angles is 50 degrees, then the corresponding angle is also 50, and the alternate interior angle is 50. The consecutive interior angle would be 130, since 50 + 130 = 180.So if ( x ) is the consecutive interior angle, it would be 130. Alternatively, if ( x ) is the corresponding angle, it would be 50.But since 130 is an option, and 50 is also an option, it's hard to say without the diagram.Wait, another idea: sometimes in these problems, you have multiple angles given, and you have to use the properties of triangles or parallel lines to find ( x ). For example, if there's a triangle with one angle given, and an exterior angle, you can find the other angles.Alternatively, if it's a problem involving the sum of angles around a point, which is 360 degrees, and some angles are given, you can subtract to find ( x ).Wait, maybe I can think of the options. 50, 60, 70, 130, 230. 50, 60, 70 are all less than 90 or 90-180. 130 is obtuse, and 230 is reflex.If ( x ) is an angle inside a triangle, it can't be 230, because triangle angles are less than 180. So if ( x ) is inside a triangle, it's either 50, 60, 70, or 130. But 130 is possible if it's an obtuse triangle.Alternatively, if ( x ) is an exterior angle, it could be 130 or 230. But 230 is a reflex angle, which is more than 180, so that would be unusual for an exterior angle.Wait, another thought: maybe ( x ) is the angle between two lines, and it's a reflex angle, so 230. But that seems a bit large.Alternatively, maybe ( x ) is part of a straight line, so it's 180 minus another angle. For example, if another angle is 50, then ( x ) is 130.Wait, let me try to think of the most common problems. If two lines intersect, forming vertical angles, and one angle is 50, then the opposite angle is 50, and the adjacent angles are 130. So if ( x ) is one of the adjacent angles, it's 130.Alternatively, if ( x ) is the reflex angle, it's 230.But which one is more likely? In most problems, unless specified, they usually ask for the smaller angle, so 130 instead of 230. But 230 is also an option.Alternatively, maybe it's a problem involving a triangle with an exterior angle. If the exterior angle is 130, then the two remote interior angles add up to 130. If one of them is 50, the other would be 80, but 80 isn't an option. Alternatively, if the remote interior angles are 60 and 70, their sum is 130, so the exterior angle is 130.So if the exterior angle is 130, then ( x ) could be 130.Alternatively, if ( x ) is the reflex angle, it's 230.Wait, but in the options, 130 is an option, and 230 is also an option. So depending on the diagram, it could be either.Wait, another approach: maybe the diagram has a triangle with angles 50, 60, and 70, and ( x ) is the exterior angle, which would be 130. So that would make ( x = 130 ).Alternatively, if ( x ) is the reflex angle outside the triangle, it would be 230.But without seeing the diagram, it's hard to know.Wait, maybe I can think of the sum of angles in a quadrilateral. If ( AB ) and ( CD ) are two sides of a quadrilateral, and some angles are given, then the sum of interior angles is 360. So if three angles are given, ( x ) can be found by subtracting from 360.But since I don't have the specific angles, it's hard to say.Wait, another thought: maybe it's a problem involving angles formed by intersecting lines and a transversal, with some given angles, and ( x ) is found using the properties of parallel lines.Wait, perhaps the diagram has two intersecting lines forming an angle of 50 degrees, and then another line creating a triangle with angles 50, 60, and 70, making ( x ) equal to 70.Alternatively, if it's a problem with parallel lines and a transversal, and one angle is 50, then the corresponding angle is 50, and the consecutive interior angle is 130.Wait, another idea: maybe it's a problem where ( x ) is the sum of two angles, like 50 and 60, making ( x = 110 ), but that's not an option. Alternatively, 50 and 70 make 120, not an option. 60 and 70 make 130, which is an option.So if ( x ) is the sum of two angles, 60 and 70, then ( x = 130 ).Alternatively, if ( x ) is the reflex angle, it's 230.Wait, maybe the diagram has a triangle with angles 50, 60, and 70, and ( x ) is the exterior angle at the 70-degree angle, making ( x = 50 + 60 = 110 ), but that's not an option. Alternatively, if ( x ) is the exterior angle at the 60-degree angle, it would be 50 + 70 = 120, not an option. If it's the exterior angle at the 50-degree angle, it's 60 + 70 = 130, which is an option.So if ( x ) is the exterior angle at the 50-degree angle, it's 130. That seems plausible.Alternatively, if ( x ) is the reflex angle, it's 230.Wait, but in most cases, unless specified, they ask for the smaller angle, so 130 instead of 230.Alternatively, maybe the diagram has a straight line with angles adding up to 180, and one angle is 50, so the adjacent angle is 130, making ( x = 130 ).Alternatively, if the diagram has a triangle with angles 50, 60, and 70, and ( x ) is one of the angles, it could be 70.Wait, but 70 is also an option. So how do I decide?Wait, maybe I can think of the most common answer. If it's a problem with two intersecting lines, and one angle is 50, then the opposite angle is 50, and the adjacent angles are 130. So if ( x ) is one of the adjacent angles, it's 130.Alternatively, if it's a triangle with angles 50, 60, and 70, and ( x ) is the exterior angle, it's 130.Alternatively, if it's a problem with parallel lines and a transversal, and one angle is 50, the consecutive interior angle is 130.So, considering all these possibilities, 130 seems like a likely answer.But wait, 230 is also an option. If the diagram shows a reflex angle, then 230 would be the answer. But without seeing the diagram, it's hard to know.Wait, another thought: maybe the diagram has a triangle with an angle of 50 degrees, and an exterior angle of 130, making ( x = 130 ).Alternatively, if the diagram has a straight line with angles 50 and 130, making ( x = 130 ).Wait, I think I need to make a decision here. Based on the options and common problems, I think ( x ) is likely 130 degrees, which is option (D).But wait, let me think again. If the diagram has two intersecting lines, forming vertical angles, and one angle is 50, then the opposite angle is 50, and the adjacent angles are 130. So if ( x ) is one of the adjacent angles, it's 130.Alternatively, if the diagram has a triangle with angles 50, 60, and 70, and ( x ) is the exterior angle at the 50-degree angle, it's 130.Alternatively, if it's a problem with parallel lines and a transversal, and one angle is 50, the consecutive interior angle is 130.So, considering all these, I think 130 is the most likely answer.Wait, but 230 is also possible if ( x ) is a reflex angle. But reflex angles are less common in basic geometry problems unless specified.So, given that, I think the answer is 130 degrees, which is option (D).But wait, let me think again. If the diagram has a triangle with angles 50, 60, and 70, then the exterior angle at the 50-degree angle is 130, which is option (D). Alternatively, if the diagram has a straight line with angles 50 and 130, making ( x = 130 ).Alternatively, if the diagram has two intersecting lines with one angle 50, then the adjacent angle is 130.So, yeah, I think 130 is the answer.Wait, but let me check if 70 is possible. If the diagram has a triangle with angles 50, 60, and 70, then ( x ) could be 70. But unless the diagram specifically points to 70, I'm not sure.Alternatively, if it's a problem with parallel lines and a transversal, and one angle is 70, then the corresponding angle is 70, and the consecutive interior angle is 110, which isn't an option.Wait, another thought: maybe the diagram has a triangle with an exterior angle of 130, which is equal to the sum of the two remote interior angles, which could be 50 and 80, but 80 isn't an option. Alternatively, 60 and 70, which add up to 130. So if the two remote interior angles are 60 and 70, the exterior angle is 130, making ( x = 130 ).So, yeah, I think 130 is the answer.Wait, but let me think about the reflex angle again. If the diagram shows a reflex angle, which is 230, then that's also possible. But unless the diagram specifically shows a reflex angle, I think 130 is more likely.So, after considering all these possibilities, I think the value of ( x ) is 130 degrees, which is option (D).**Final Answer**boxed{D}
question:A dedicated gymnast is preparing for an upcoming championship and has a specific training routine that involves both strength and flexibility exercises. She has observed that the number of strength exercises, ( s ), she performs is always twice the square of the number of flexibility exercises, ( f ), she does. Additionally, her total training time is limited to 6 hours per day. Each strength exercise takes 20 minutes, and each flexibility exercise takes 15 minutes.1. Write an equation relating the number of strength exercises and flexibility exercises she performs in a day and determine the possible number of flexibility exercises she can include in her routine.2. If her goal is to maximize the number of flexibility exercises while still adhering to her total training time constraint, how many flexibility exercises should she perform, and how many strength exercises will that correspond to?
answer:First, I need to establish the relationship between the number of strength exercises (( s )) and flexibility exercises (( f )). According to the problem, the number of strength exercises is twice the square of the number of flexibility exercises. This gives me the equation:[ s = 2f^2 ]Next, I need to consider the total training time constraint. Each strength exercise takes 20 minutes, and each flexibility exercise takes 15 minutes. The total time spent on exercises should not exceed 6 hours, which is 360 minutes. This leads to the time constraint equation:[ 20s + 15f leq 360 ]Substituting the expression for ( s ) from the first equation into the time constraint equation:[ 20(2f^2) + 15f leq 360 ][ 40f^2 + 15f - 360 leq 0 ]To simplify, I'll divide the entire equation by 5:[ 8f^2 + 3f - 72 leq 0 ]Now, I need to solve this quadratic inequality. First, I'll find the roots of the equation ( 8f^2 + 3f - 72 = 0 ) using the quadratic formula:[ f = frac{-b pm sqrt{b^2 - 4ac}}{2a} ][ f = frac{-3 pm sqrt{9 + 2304}}{16} ][ f = frac{-3 pm sqrt{2313}}{16} ]Calculating the square root of 2313 gives approximately 48.1. Therefore, the roots are:[ f = frac{-3 + 48.1}{16} approx 2.82 ][ f = frac{-3 - 48.1}{16} approx -3.19 ]Since the number of exercises cannot be negative, I'll consider only the positive root, approximately 2.82. The quadratic inequality ( 8f^2 + 3f - 72 leq 0 ) holds between the roots, but since ( f ) must be a non-negative integer, the possible values for ( f ) are 0, 1, 2, and 3.Finally, to maximize the number of flexibility exercises while staying within the time constraint, the gymnast should perform 3 flexibility exercises. Substituting ( f = 3 ) back into the equation for ( s ):[ s = 2(3)^2 = 18 ]So, she should perform 3 flexibility exercises and 18 strength exercises.